[TIL] if문 예제 풀이
문제 풀이 )
1)
큰수를 먼저 if문으로 판별하여 차이를 출력하는 방법이다.
이외에 먼저빼고 음수 양수 여부에 따라 부호를 바꾸는 방법도 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
package ex0723;
import java.util.Scanner;
public class ifTest1 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a, b, c;
System.out.print("첫번째 수?");
a = sc.nextInt();
System.out.print("두번째 수?");
b = sc.nextInt();
if (a > b) {
c = a - b;
} else {
c = b - a;
}
// 다른 방법
// c = a - b;
// if(c>0){
//c = -c;
//}
System.out.println("두수의 차이?" + c);
sc.close();
}
}
|
cs |
문제풀이
2)
논리곱과 논리합 연산자를 if 문 조건식에 대입하여 푼 문제다.
여기서 수정해야할 점은 print 메서드는 되도록이면 마지막에 넣어주는게 바람직하다.
if문안에는 되도록이면 삼가는게 좋다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
package ex0723;
import java.util.Scanner;
public class ifTest2 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int year;
System.out.print("년도?");
year = sc.nextInt();
if(year%4==0 && year%100!=0 ||year%400==0) {
System.out.println(year +"년도는 윤년입니다.");
}else {
System.out.println(year +"년도는 평년입니다.");
}
sc.close();
}
}
|
cs |
문제풀이
3)
문자의 아스키 코드를 활용하여 대소문자롤 변경하는 문제다.
논리곱의 연산과 강제 형변환을 활용하여 int형을 char형으로 변환하였다.
여기서도 마찬가지로 print 메서드는 되도록 마지막에 작성하자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
|
package ex0723;
import java.util.Scanner;
public class ifTest3 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
char a,b;
System.out.print("문자?");
a = sc.next().charAt(0);
if(97<=a&&a<=122) {
b= (char)(a-32);
System.out.println(a +"->" +b);
}else if(65<=a&&a<=90) {
b= (char)(a+32);
System.out.println(a +"->" +b);
}else {
System.out.println(a +"->" +a);
}
sc.close();
}
}
|
cs |
문제풀이
4)
else if문을 활용하여 점수대 별 다른 점수를 출력하는 제어문을 작성했다.
한가지 실수 한점은 점수가 0~100점이 아닌 다른 점수를 입력시 입력 오류가 나오는 것을 빼먹었다.
이를 수행하기 위해서는 if문 처음에 점수 범위를 먼저 판별하여 else에 입력 오류 출력을 삽입해야한다.
그리고 또한가지 실수한점은
첫 if문 조건식에서 큰수부터 내려오면 굳이 논리곱을 활용하여 길게 조건식을 작성할 필요가없다.
이미 윗 조건식에서 걸러서 오기때문에 이점을 꼭 유념해야한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
|
package ex0723;
import java.util.Scanner;
public class ifTest4 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a;
double b;
System.out.print("점수?");
a = sc.nextInt();
if (95 <= a && a <= 100) {
b = 4.5;
} else if (90 <= a && a <= 94) {
b = 4.0;
} else if (85 <= a && a <= 89) {
b = 3.5;
} else if (80 <= a && a <= 84) {
b = 3.0;
} else if (75 <= a && a <= 79) {
b = 2.5;
} else if (70 <= a && a <= 74) {
b = 2.0;
} else if (65 <= a && a <= 69) {
b = 1.5;
} else if (60 <= a && a <= 64) {
b = 1.0;
} else {
b = 0.0;
}
/*
* if(a>=0&&a<=100) 으로 범위내로 정하고 아래부분은 and연산 넣지않기
* if(a>=95) b=4.5;
* else if(a>=90) b=4.0;
* else if(a> 등등)
*/
System.out.printf("점수 : %d , 평점 : %.1f", a,b);
sc.close();
}
}
|
cs |
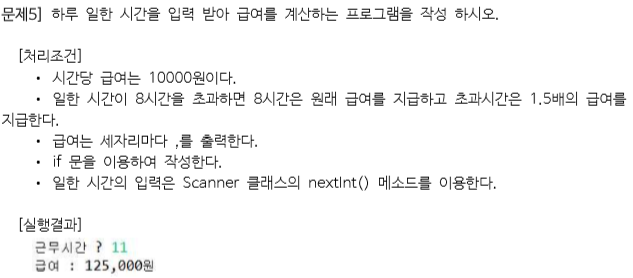
문제풀이
5)
if문을 통하여 급여 계산을 해보았다.
여기서 유의할 점은 시간과 급여가 int형으로 선언이 되어있기때문에 초과시간 페이를 계산하고나서
강제로 int형으로 형변환을 해줘야 오류가 없다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
|
package ex0723;
import java.util.Scanner;
public class ifTest5 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int hour, pay;
System.out.print("근무시간");
hour = sc.nextInt();
if(hour>8) {
pay = (int)(8*10000 + (hour-8)*(10000*1.5));
}else {
pay = hour*10000;
}
System.out.printf("급여 :%,d원",pay);
sc.close();
}
}
|
cs |

문제풀이
6)
처음에 좀 막혔던 문제이다.
연산자 기호를 아스키코드로 인식하고 변환하는게 제일 중요했던 문제다.
그리고 else if문 사용시 괄호없이 줄을 이어서 작성해도 된다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
|
package ex0723;
import java.util.Scanner;
public class ifTest6 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a, b, d;
char c;
System.out.print("두수?");
a = sc.nextInt();
b = sc.nextInt();
System.out.print("연산자[+-*/]?");
c = sc.next().charAt(0);
if (c == 42) {
d = a * b;
System.out.printf("%d * %d = %d", a, b, d);
} else if (c == 43) {
d = a + b;
System.out.printf("%d + %d = %d", a, b, d);
} else if (c == 47) {
d = a / b;
System.out.printf("%d / %d = %d", a, b, d);
} else if (c == 45) {
d = a - b;
System.out.printf("%d - %d = %d", a, b, d);
}
sc.close();
}
}
|
cs |
문제풀이
7)
논리곱을 3개를 사용하여 3가지 조건을 만족하는 조건식을 작성했다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
package ex0723;
import java.util.Scanner;
public class ifTest7 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a, b, c;
String name;
System.out.print("이름?");
name = sc.next();
System.out.print("세과목 점수?");
a = sc.nextInt();
b = sc.nextInt();
c = sc.nextInt();
if (a >= 40 && b >= 40 && c >= 40 && (a + b + c) / 3 >= 60) {
System.out.printf("%s님은 합격입니다.", name);
} else if(a+b+c<60) {
System.out.printf("%s님은 불합격입니다.", name);
}else {
System.out.printf("%s님은 과락입니다.", name);
}
sc.close();
}
}
|
cs |

문제풀이
8)
마지막 문제답게 조금은 길고 헷갈렸던 문제다.
변수 선언이 많아서 주석으로 내용을 정리하고 작성하는게 중요하다.
여기서 주의할점은 학번과 같은 번호로된 정보는 int형같은 자료형에 선언하지 않는게 좋다.
번호를 숫자로 인식하기 때문에 번호가 손실될 가능성이 높고 추후에 사용될때 어려움이 될수 있다. 되도록이면 String 을 사용하자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
|
package ex0723;
import java.util.Scanner;
public class ifTest8 {
public static void main(String[] args) {
Scanner sc=new Scanner(System.in);
String hak, name;
int s1, s2;
int a, r;
int midterms, finals;
int attend, report;
int score;
char grade;
System.out.print("학번?");
hak=sc.next();
System.out.print("이름?");
name=sc.next();
System.out.print("중간고사점수?");
s1=sc.nextInt();
System.out.print("기말고사점수?");
s2=sc.nextInt();
System.out.print("결석횟수?");
a=sc.nextInt();
System.out.print("리포트점수?");
r=sc.nextInt();
// 중간 및 기말 환산 점수
midterms=(int)(s1*0.4); // 중간고사
finals=(int)(s2*0.4); // 기말고사
// 출석점수
if(a>=6) attend=0;
else if(a>=4) attend=60;
else if(a>=2) attend=80;
else attend=100;
// 출석 환산 점수
attend = (int)(attend*0.1);
// 리포트 환산 점수
report = (int)(r*0.1);
// 총 합산 점수
score = midterms+finals+attend+report;
// 학점
if(score>=90) grade='A';
else if(score>=80) grade='B';
else if(score>=70) grade='C';
else if(score>=60) grade='D';
else grade='F';
// 출력
System.out.print("\n학번\t이름\t");
System.out.print("중간\t기말\t");
System.out.print("출석\t레포트\t");
System.out.print("합산\t학점\n");
System.out.print(hak+"\t");
System.out.print(name+"\t");
System.out.print(s1+"\t");
System.out.print(s2+"\t");
System.out.print(attend+"\t");
System.out.print(report+"\t");
System.out.print(score+"\t");
System.out.print(grade+"\n");
sc.close();
}
}
|
cs |
'Language > JAVA' 카테고리의 다른 글
[TIL] while 문 (0) | 2020.07.24 |
---|---|
[TIL]switch - case 문 (0) | 2020.07.24 |
[TIL] if문 (0) | 2020.07.23 |
[TIL]형 변환 (0) | 2020.07.23 |
[TIL] 비트 단위 연산자 (0) | 2020.07.23 |
댓글