[TIL] 비트 단위 연산자
오늘 배운 내용은 비트 단위 연산자 bitwise operators
byte,short,char 타입은 자동으로 int로 변환된다.
boolean은 비트 단위 shift연산을 제외하고 가능하다.
double,float 형은 사용할 수 없다.
비트 단위 부정 연산자
정수형 자료의 컴퓨터 내부 2진수 값을 1의 보수(반전)된 값을 구하는 단항 연산자
비트 단위 논리 연산자
정수형 자료의 내부 2진수 값을 대상으로 논리 연산을 수행하는 이항 연산자
비트 단위 논리 연산자는 단축연산을 하지 않는다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
|
package ex0723;
public class BitEx1 {
public static void main(String[] args) {
int a =12, b=6;
int c;
// 12 : 0000 1100 (2진수)
// 6 : 0000 0110 (2진수)
c=~b; //6 반전 값 =-7
System.out.println(c);
// 12 : 0000 1100 (2진수)
// 6 : 0000 0110 (2진수)
// 0000 0100
c=a&b; //4
System.out.println(c);
// 12 : 0000 1100 (2진수)
// 6 : 0000 0110 (2진수)
// 0000 1110
c=a|b; //14
System.out.println(c);
// 12 : 0000 1100 (2진수)
// 6 : 0000 0110 (2진수)
// 0000 1010
c=a^b; //10
System.out.println(c);
}
}
|
cs |
변수의 변환없이 값 바꾸기가 가능하다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
package ex0723;
public class BitEx2 {
public static void main(String[] args) {
int a =12, b=6;
/*int c;
c=a;a=b;b=c;
*/
//변수없이 값 바꾸기가 가능함
a=a^b;
b=b^a;
a=a^b;
System.out.println(a+","+b);
}
}
|
cs |
논리 연산을 통해 짝수 홀수 판별도 가능하다. 연산시 연산자 우선순위를 고려해야한다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
package ex0723;
public class BitEx3 {
public static void main(String[] args) {
int a ;
String s;
a=13;
// s = a%2 == 0 ? "짝수": "홀수";
// 10 ; 0000 1010 => 짝수는 마지막 자리가 무조건 0
// 13 : 0000 1101
// 1 : 0000 0001
//a&1 : 0000 0001 // 끝자리가 홀수면 1 짝수면 0
s = (a&1) == 1 ? "홀수":"짝수";
// &연산자보다 == 연산자의 우선순위가 더 높으므로 괄호를 해줘야함 System.out.println(s);
}
}
|
cs |
비트 논리 연산자로 암호화와 복화화를 할 수 있다.(기본적인)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
|
package ex0723;
public class BitEx4 {
public static void main(String[] args) {
int a =13, b = 10;
//13 : 0000 1101
//10 : 0000 1010
//a^b: 0000 0111
/// 0000 1010 7
// 0000 1101 13
a=a^b; // encryption // 7 =>암호화 시 기본 연산
System.out.println(a);
a=a^b; // decryption // 13 =>복화화의 기본
System.out.println(a);
}
}
|
cs |
비트 단위 이동 연산자
정수형 자료의 내부 비트를 좌측 또는 우측으로 이동 시키는 이항 연산자
주로 암호화 알고리즘에 사용된다.
우측으로 이동하면 2배 4배 8배 만큼 줄어든다 이는 즉: 나눗셈이 가능하다는 말
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
|
package ex0723;
public class BitEx5 {
public static void main(String[] args) {
int a ,b;
// 비트 우측 shift 연산
a= 128;
b=a>>3;
// 128을 2의 3승으로 나눈 것과 같음
// 우측에 남는 자리는 부호로 채운다.
System.out.println(b);//16
// 비트 좌측 shift 연산
b=a<<3;
//좌측의 남는 자리는 0으로 채움
//a를 2의 3승으로 곱한 값과 동일
System.out.println(b);//1024
a =-16;
b=a>>>3;
//남는 자리는 무조건 0으로 채움 => 부호가 양수가 되면서 수가 커짐
System.out.println(b); //536870910
}
}
|
cs |
고난도 : 숫자 로테이션
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
|
package ex0723;
import java.util.Scanner;
public class BitEx6 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int a, offset;
int temp, result;
System.out.print("정수?");
a = sc.nextInt();
System.out.print("이동자리수?");
offset = sc.nextInt();
temp = a >>> (32-offset);
result = a<< offset;
result = result | temp;
System.out.printf("수:%d(%#X)\n",a,a);
System.out.println("왼쪽이동자리수"+offset);
System.out.printf("결과:%d(%#X)\n", result, result);
sc.close();
}
}
// 수 : 1011 1000
//<- 방향으로 2칸 이동
//2bit를 왼쪽으로 로테이트 : 1110 0010
// temp = a >>> (8-offset); // 우측으로 6칸 이동 빈칸은 0으로 채움
// 0000 0010
// result = a << offset;
// 1110 0000
//result = result | temp;
//1110 0000
//0000 0010
//1110 0010 // 로테이트 시킴
|
cs |
연산 우선순의 주의 ~ > & > ^ < |
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
package ex0723;
public class BitEx6 {
public static void main(String[] args) {
byte x =0x0a, y=0x48;
int z = (byte) 0xcc;
int a;
// x : 0000 1010 10
// y : 0100 1000 72
// z : 1100 1100 -52
// 연산 우선순위 : ~ > & > ^ < |
a = (byte)(x | y & z);
System.out.println(a); //74
a = (byte)(x | y & ~z);
System.out.println(a);
a = (byte)(x ^ y & ~z);
System.out.println(a);
}
}
|
cs |
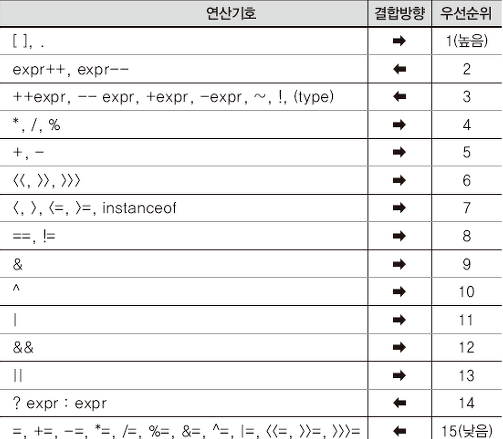
'Language > JAVA' 카테고리의 다른 글
[TIL] if문 (0) | 2020.07.23 |
---|---|
[TIL]형 변환 (0) | 2020.07.23 |
[TIL] printf()메서드의 관한 서식 (0) | 2020.07.22 |
[TIL]배정연산자(+=,-=,*=,/= 등등) (0) | 2020.07.22 |
[TIL]조건연산자(삼항연산자) (0) | 2020.07.22 |
댓글